Sponsor: Using RabbitMQ or Azure Service Bus in your .NET systems? Well, you could just use their SDKs and roll your own serialization, routing, outbox, retries, and telemetry. I mean, seriously, how hard could it be?
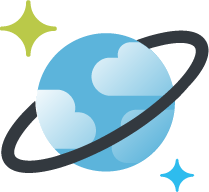
Concurrency
The first thing I needed to implement was how to handle concurrency. Specifically optimistic concurrency.In an optimistic concurrency model, a violation is considered to have occurred if, after a user receives a value from the database, another user modifies the value before the first user has attempted to modify it.This is pretty typical when dealing with multi user environments like a web application. Specifically in my case is:
- Fetching out a document from Azure Cosmos DB
- Mutating the data of the document
- Sending the document back to to Azure Cosmos DB to be replaced
ETags
Each document within Azure Cosmos DB has an ETag Property.The ETag or entity tag is part of HTTP, the protocol for the World Wide Web. It is one of several mechanisms that HTTP provides for web cache validation, which allows a client to make conditional requests.You may be familiar with ETag’s related caching. A typical scenario is a user makes an HTTP request to the server for a specific resource. The server will return the response along with an ETag in the response header. The client then caches the response along with the associated ETag.
ETag: "686897696a7c876b7e"If they client then makes another request to the same resource, it will pass a If-Non-Match header with the ETag it received.
If-None-Match: "686897696a7c876b7e"If the resource has not changed and the ETag represents the current version, then the server will return a 304 Not modified status. If the resource has been modified, it will return the appropriate 2XX status code along with the content new ETag header.
AccessCondition
Azure Cosmos DB uses ETags for handling optimistic concurrency. When we retrieve a document from Azure Cosmos DB, it always contains an ETag property as apart of our document. When we then want to send our request to replace a document, we can specify anAccessCondition
with the ETag we received when we fetched out our document.
If the ETag we send is not current, the server will return a 412 Precondition Failed status code. In our .NET SDK, this is wrapped up in a DocumentClientException
.
Here is a full an example.
Demo Source Code
I’ve put together a small .NET Core sample with an XUnit test from above. All the source code for this series is available on GitHub.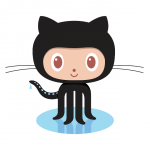